URL validation: Use PHP to format and validate a URL with these functions!
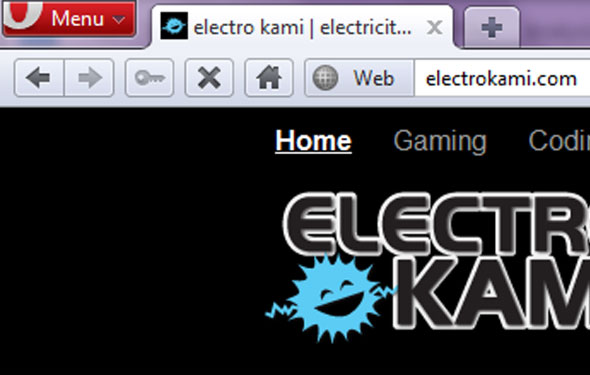
The only URL that you need to know 🙂
Have you ever needed to validate or format a website URL with PHP and found that the PHP function parse_url() doesn’t quite do all that you need it to?
Look no further! Here is a set of functions that you can use to format, validate, and “clean up” URLs that come from user input, databases, or even variables. It is the easiest way for you to run your URLs through validation.
All you have to do is call this function:
[php]
<?php
$urlToCompute = nicifyUrl($url);
?>
[/php]
And the custom functions take care of the rest! You will find this useful if you have a contact form or some kind of cool web script that requires a user-input field for website.
Check out the full set of free to use functions below along with a simple example to show you how you can use these validators and formatters. I’d recommend sticking them into a class to keep your code clean!
[php]
<?php
// get url to check from the page parameter ‘url’
// or use default http://example.com
$url = isset($_GET[‘url’])
? $_GET[‘url’]
: "http://example.com";
// put functions to work
$urlToCompute = nicifyUrl($url);
// show result
if($urlToCompute == "Invalid URL"){
echo $_GET[‘url’] . " is not a valid URL";
}
else{
echo $urlToCompute . " is a valid URL";
}
// functions
function nicifyUrl($url) {
$url = urldecode(strtolower($url));
$url = stripit($url);
// clean up url path
$url = explode("/",$url);
$arrUrl = parse_url($url[0]);
$urlRet = $arrUrl[‘path’];
// valid?
if(validateTheUrl($urlRet)){
return $urlRet;
}
else{
return "Invalid URL";
}
}
function stripit($url){
$url = trim($url);
$url = preg_replace("/^(http:\/\/)*(www.)*/is", "", $url);
$url = preg_replace("/\/.*$/is" , "" ,$url);
return $url;
}
function validateTheUrl($url){
if (!preg_match("#[a-z0-9-_.]+\.[a-z]{2,4}#i",$url)) {
return false;
}
else if(strpos($url,"@") !== false){
return false;
}
else{
return true;
}
}
?>
[/php]
This will also detect sub-domains and mark them as valid, along with a few other types of uncommon urls.
And, as mentioned before, these are free to use and change – do whatever you want with them! As long as it makes your website better, it makes me happy!