A cool PHP tip to make your programs more efficient: Associative Array Logic
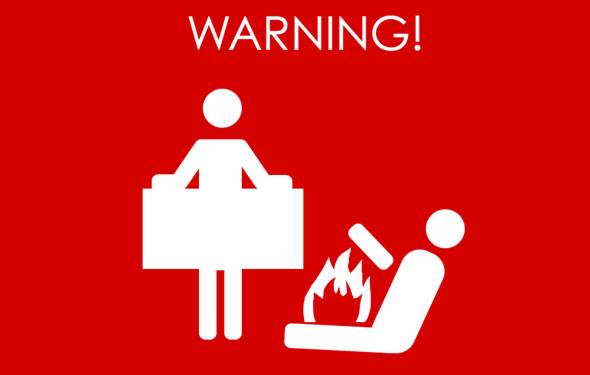
Note: This script will not allow you to combust things. Not yet at least.
Do you have a lot of data that is being called with the help of if statements?
Or perhaps you have a massive switch statement that is being utilized to define or populate certain variables or settings?
Here is a quick and dirty PHP tip that you might be able to use within one of your next projects.
As an extra plus, it will also work in practically any other programming language that allows associative arrays.
The need for some logic
Say you have a need for some more logic to wrap around our jQuery Style Sheet Switcher using PHP.
Or maybe you have a cool time-of-day based CSS switch that is controlled by PHP code and lets your site visitors see different themes depending on the server time.
There are multiple ways to solve this logic problem. Where do we begin?
You could try a large amount of if->else statements:
[php]
<?php
$styleToShow = getTimeOfDay();
// this variable would be set
// using a function that would
// return a string value
if($styleToShow == "default"){
echo "styles.css" . "<br />";
}
else if($styleToShow == "noon"){
echo "noon.css" . "<br />";
}
else if($styleToShow == "night"){
echo "night.css" . "<br />";
}
?>
[/php]
Or likewise you could use the trusty old switch statement for some cleaner block logic:
[php]
<?php
$styleToShow = "night";
// this variable would be set
// using a function that would
// return a string value
switch($styleToShow){
case "default":
echo "styles.css" . "<br />";
break;
case "morning":
echo "morning.css" . "<br />";
break;
case "noon":
echo "noon.css" . "<br />";
break;
case "night":
echo "night.css" . "<br />";
break;
}
?>
[/php]
Using an associative array for if, else, or switch logic
But we have yet another method that utilizes an associative array and allows a much easier to read code block and quicker logic.
Take a look at this cool logic:
[php]
<?php
$styleToShow = array(
"default" => "styles.css",
"morning" => "morning.css",
"noon" => "noon.css",
"afternoon" => "afternoon.css",
"night" => "night.css"
);
echo $styleToShow["default"] . "<br />";
echo $styleToShow["morning"] . "<br />";
// this variable could be
// set from a function
$theTime = "night";
echo $styleToShow[$theTime] . "<br />";
?>
[/php]
With this method, you have a global variable that can be set in your class variables or config file and will allow a much easier method to make any changes to stylesheet names or logic triggers.
As a matter of fact, this method also makes it easier to change the associative array to allow even more data (or different data) without any extra coding:
[php]
<?php
$styleToShow = array(
"default" => "styles.css",
"1am" => "1am.css",
"2am" => "2am.css",
"3am" => "3am.css",
"4am" => "4am.css",
"5am" => "5am.css"
);
echo $styleToShow[$theTime] . "<br />";
?>
[/php]
You just made your PHP code much more portable and easier to modify!
Congratulations!